What is variable?
What is a variable?
A variable is a storage for a value, which can be a string, a number, or something else. Every variable has a name (or an identifier) to distinguish it from other variables. You can access a value by the name of the variable.
Declaring variables
Before you can start using a variable, you must declare it. To declare a variable, Kotlin provides two keywords:
val (for value) declares a read-only variable (just a named value), which cannot be changed after it has been initialized, so a value can be assigned once (this is actually not entirely true, we will discuss this issue in more detail later);
var (for variable) declares a mutable variable, which can be changed (as many times as needed).
const is used for variables (with val) that are known at compile-time.
var and val are both used to declare variables in Kotlin language.
VAR(Variable)
var is the general variable, and it was assigned multiple times also it is known as a mutable variable in a kotlin.
What is var?
In kotlin language var is used to declare the variables. Var is a general variable defined in the language of kotlin. The variable value is declared using var, and we can change the same throughout the program. In kotlin language var is also called as non-final variable and mutable, as we can change the var value anytime in a program.
Below example shows kotlin var as follows. In the below example we can see that it will display the output of current age as well as after 2 days age. So we can say that we can change the value at any time in the program by using kotlin var. In below example first we are setting the age is 10 then we are setting the age is 11 then in our output both value will be displayed.
//Example
fun main()
{
var age = 10
println("Current age is: $age")
age = 11
println("After 2 days age is : $age")
}
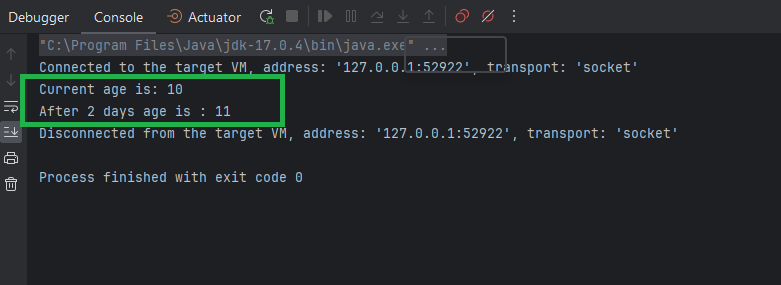
VAL(Value)
val is the constant variable which was assigned multiple times and which was initialized in a single time and it is also known as variable of immutable in kotlin.
What is val?
The object which we are storing by using val is not changed; we cannot reassign.
val is immutable, so once we have assigned the val, it will become read-only. The property of val object is changed, but the object is read-only.
In below example we can see that first we have defined the age is 10 years and then we have defined the age val as 11 years, it will give the error val cannot be reassigned. Basically we are using val in kotlin for declaring a variable, val is the consistent variable and which we are assigning multiple times.
//Example
fun main()
{
val age = 10
println("Current age is " + age)
age = 11
println("After 2 days age is " + age)
}
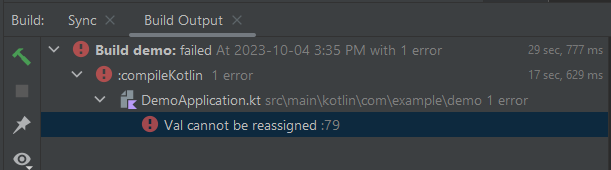
fun main()
{
val language = Language("Kotlin", "JetBrains")
println("After Assign Once : $language")
language.name = "JavaScript"
language.developer ="Brendan Eich"
println("Before Reassign : $language")
}
data class Language(
var name : String = "",
var developer : String = ""
)
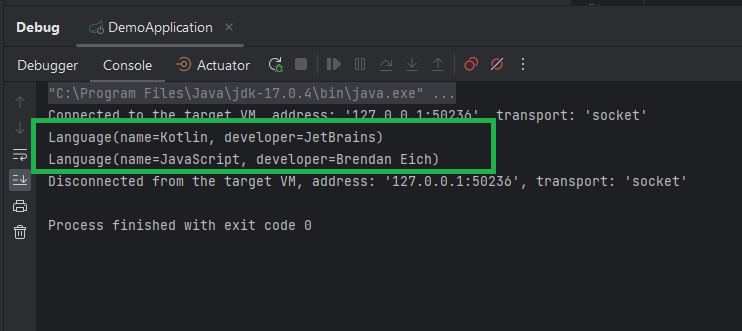