Comparison Operators In MongoDB
MongoDB uses various comparison query operators to compare the values of the documents. The following table contains the comparison query operators:
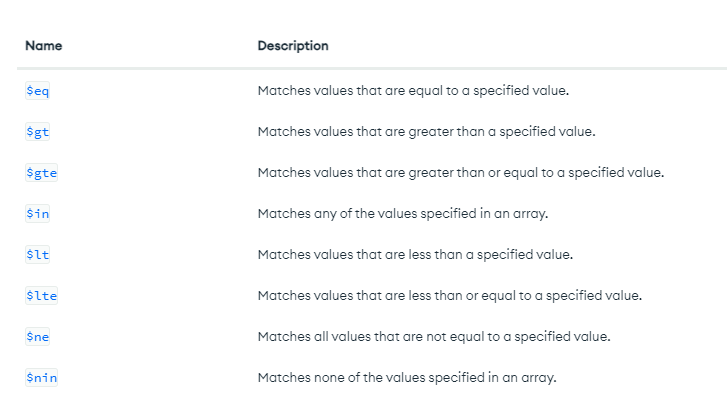
Matching values using $nin operator:
In this example, we are retrieving only those employee’s documents whose name is not Daisy or Amruta.
//Example
db.q_employee.find({name: {$nin: ["Daisy", "Amruta"]}})
Matching values using $in operator:
In this example, we are retrieving only those employee’s documents whose name is either Daisy or Amruta.
//Example
db.q_employee.find({name: {$in: ["Daisy", "Amruta"]}})
Matching values using $lt operator:
In this example, we are selecting those documents where the value of the vacations field is less than 4.
//Example
db.q_employee.find({vacations: {$lt: 4}})
Matching values using $eq operator:
In this example, we are selecting those documents where the value of the role field is equal to MANAGER.
//Example
db.q_employee.find({ROLE: {$eq: "MANAGER"}})
Matching values using $ne operator:
In this example, we are selecting those documents where the value of the role field is not equal to MANAGER.
//Example
db.q_employee.find({ROLE: {$ne: "MANAGER"}})
Matching values using $gt operator:
In this example, we are selecting those documents where the value of the vacations field is greater than 1.
//Example
db.q_employee.find({vacations: {$gt: 1}})
Matching values using $gte operator:
In this example, we are selecting those documents where the value of the vacations field is greater than equals to 2.
//Example
db.q_employee.find({vacations: {$gte: 2}})
Matching values using $lte operator:
In this example, we are selecting those documents where the value of the vacations field is less than equals to 1.
//Example
db.q_employee.find({vacations: {$lte: 1}})