Promises And Promise Chaining
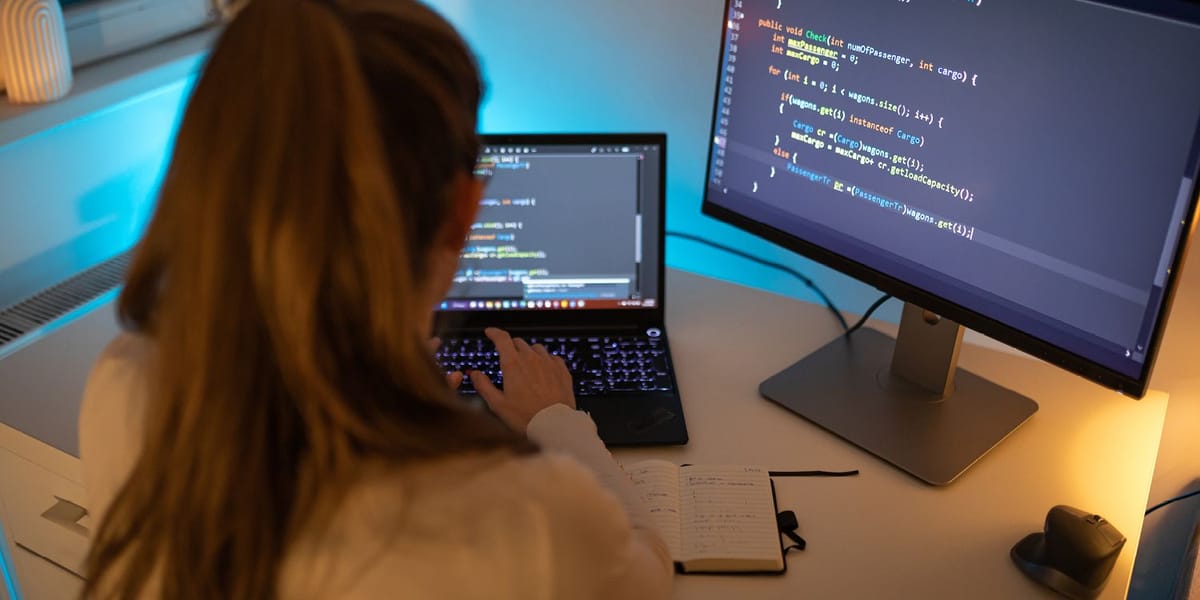
What are promises in JavaScript?
- Promise is a special object in JavaScript. Promise provides us with a value after an asynchronous operation is successfully completed, even when the operation is completed but is not successfully due to timeout error or any other type o error.
- Whenever an async operation is successful its completion is indicated by a resolve function call, and in case o an error it is indicated by a reject function call.
- A promise has three states that are known:
- Pending - It is the initial state when the execution starts.
- Fulfilled - When the promise is resolved.
- Rejected - When the promise is rejected.
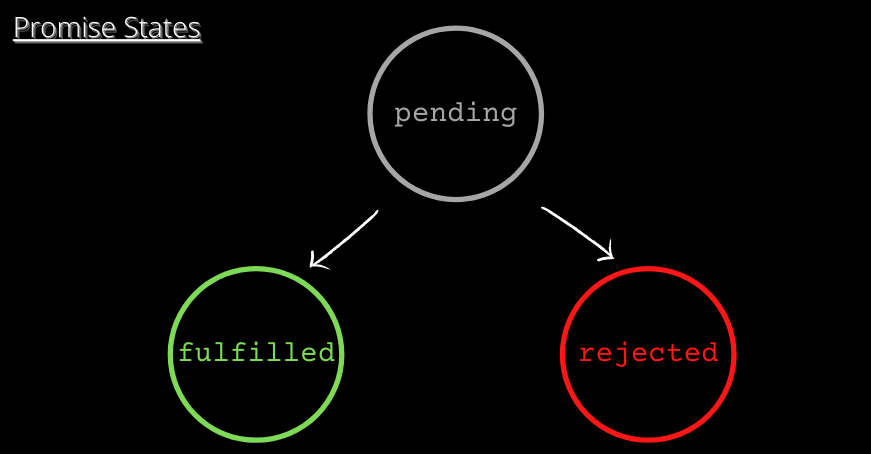
- A promise created by using a promise constructor.

- An example of a promise is given below:
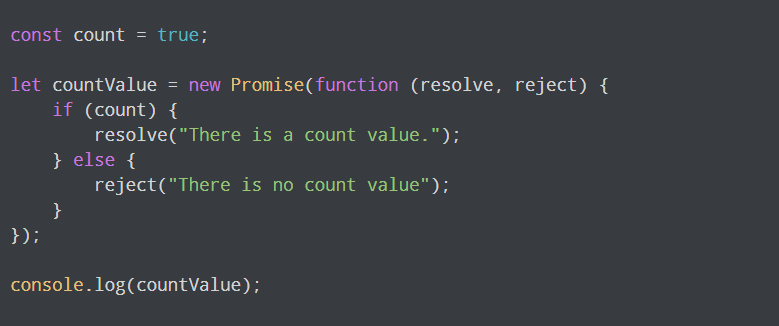
Output:

- Promise Handlers : .then() , .catch() and .finally()
Promise handlers help to create the link between the executor and the consumer functions so that they can be in sync when a promise resolve
s or reject
s.
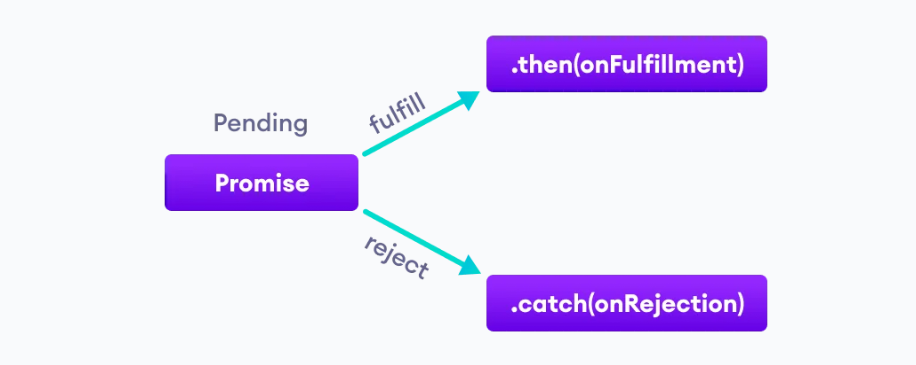
The final result in a promise can be received through .then() and .catch(). Mostly if the intended result is successful .then() is used where as or reject or an unsuccessful program .catch() is used. In promises .finally() is used to program general cleanups and is executed no matter the promise is resolved successfully or rejected.
What is Promise Chaining?
- We can chain promises together by passing the resolve value of a promise with the help of .then() method to the other promise.
- There are also handler methods that handle multiple promises.
- Promise.all() - It executes multiple promises simultaneously.
- Promise.any() - Returns a single Promise from a list of promises, when any promise fulfill.
- Promise.allSettled() - Used when you have multiple asynchronous tasks that are not dependent on one another to complete successfully, or you'd always like to know the result of each promise.
- Promise.race() - Execute multiple promises and use the result of the first one that resolves.
- Promise.resolve() - It is a static method "resolves" a given value to a Promise.
- Promise.reject() - It returns a Promise that is rejected.
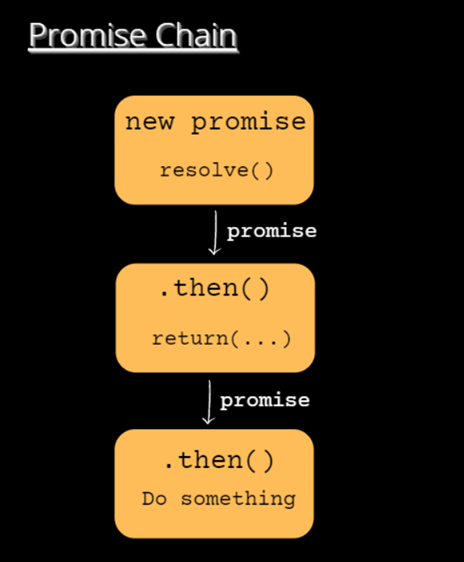
- Example:
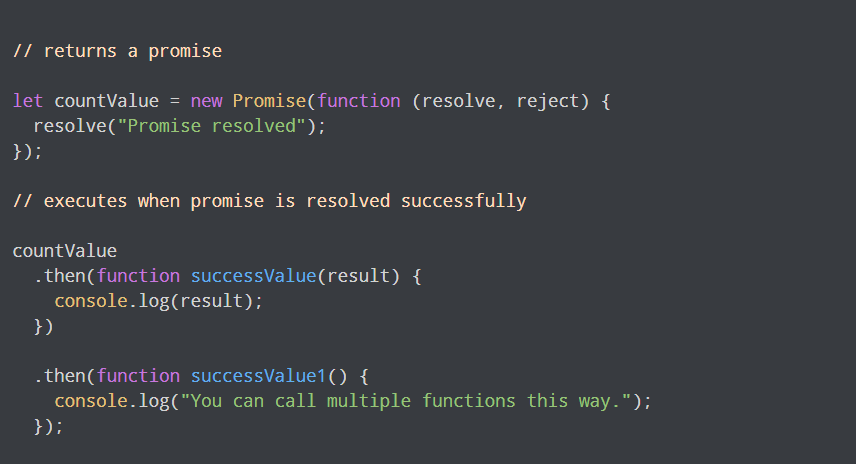
Output:
