Navigation in React JS:
- What is Navigation ?
- In React.js, navigation typically refers to the process of moving between different parts of your application based on user actions, such as clicking a link or submitting a form.
- React doesn't have built-in support for routing (navigation between different pages or views), so developers often use third-party libraries to handle this functionality.
- The most popular library for routing in React applications is React Router.
- How to handle navigation in React using React Router ?
- Installing React Router: Installation of React Router if not installed prior.

- Setting Up Routes: Setting up routes in the main 'App' component dedicated routing component (eg: RoutePathConstant ) with help of
BrowserRouter
andRoute
components provided by React Router.
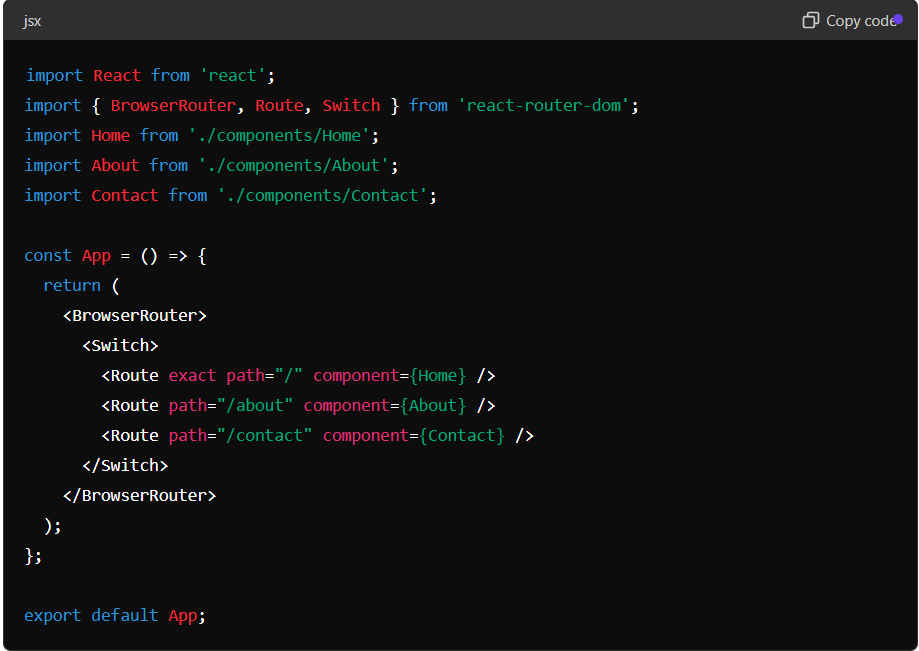
- Navigating Between Routes:
- Using Links:
Link
component provided by React Router prevents the default anchor tag behavior and uses the HTML5 history API to keep your UI in sync with the URL can be used.
- Using Links:
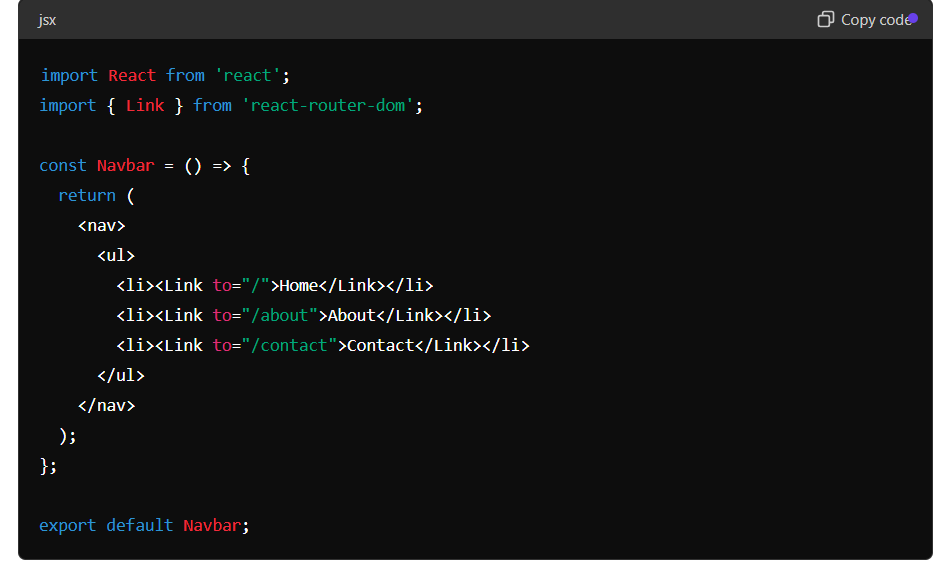
- Programmatically:
history
object provided by React Router, especially when handling form submissions or other events can be used.
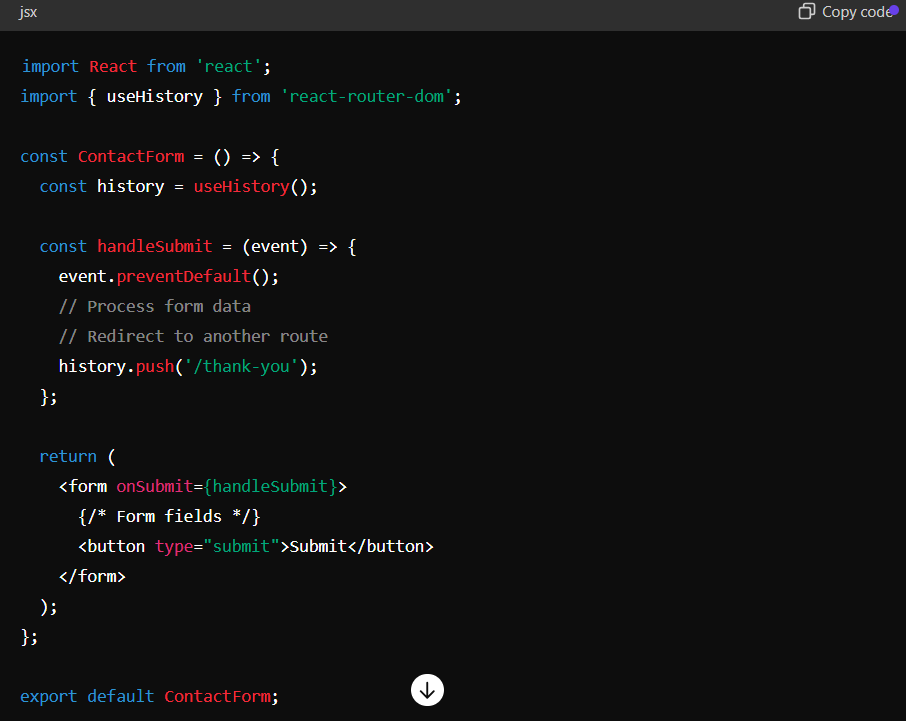
- Handling Route Parameters: If your routes require dynamic parameters (e.g.,
/users/:id
), you can use theuseParams
hook provided by React Router to access those parameters.
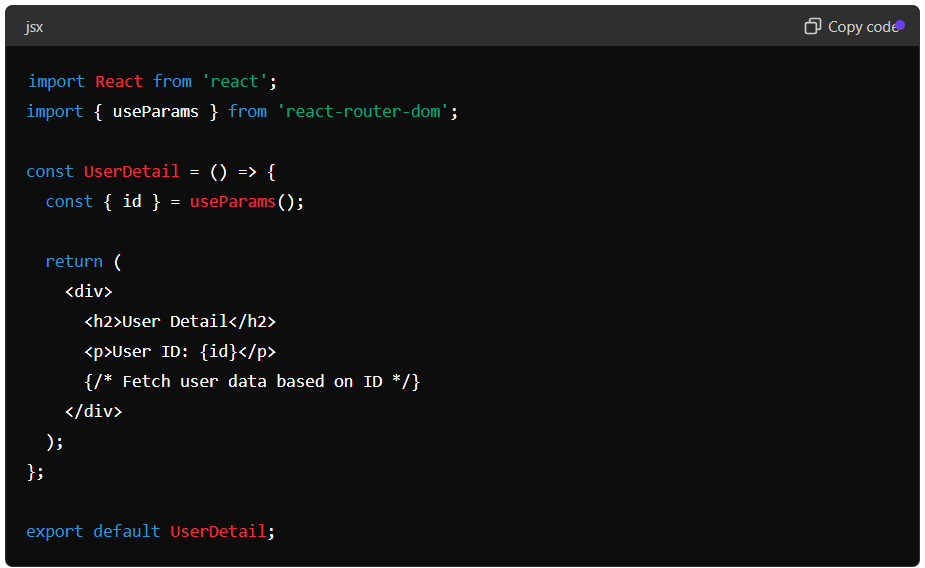
- Flowchart :
Detailed flowchart for navigation in React.js involves illustration of how components, routes, and navigation actions interact within a typical React application using React Router. Here’s a more comprehensive flowchart representation:
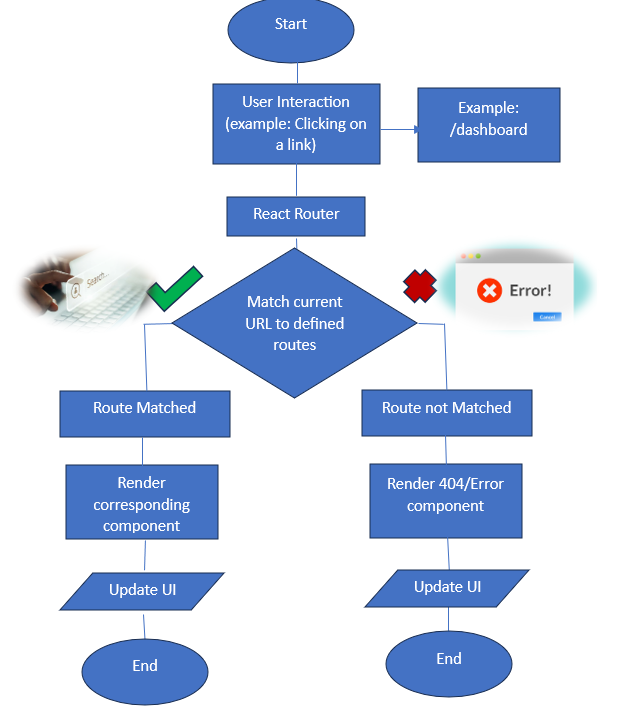
- Flowchart Explanation:
- User Interaction: The user interacts with the application, triggering a navigation event.
- React Router: The React Router library receives the navigation event.
- Match Current URL to Defined Routes: The React Router compares the current URL to the defined routes in the application. Eg: when the path is matched the corresponding component which bears that path is then rendered.
- Match Route: If the current URL matches a defined route, the corresponding component is rendered.
- Render Corresponding Component: The matched component is rendered, and the user interface is updated.
- No Match Route: If the current URL does not match any defined route, a 404 or error component is rendered.
- Render 404/Error Component: The 404 or error component is rendered, and the user interface is updated.
- Update UI: The user interface is updated to display the new content.
- End: The navigation process is complete.
- Key Points:
- React Router: Manages navigation and rendering of components based on URL changes.
- Component Lifecycle: Components may initialize, fetch data, and update based on navigation actions.
- User Interaction: Flowchart emphasizes how user actions drive navigation within the React application.
- Dynamic Routing: Illustrates how React Router dynamically updates components based on URL changes.
This flowchart provides a structured overview of the navigation flow in a React.js application, illustrating the interaction between user actions, routing definitions, component rendering, and data updates. Adjustments can be made based on specific application requirements and complexity.
- Navigating Files in a React Project
As a React developer, it's important to have a solid understanding of how to navigate and structure the files in your web project. Proper file organization can make your codebase more maintainable, scalable, and easier to work with. In this blog post, we'll explore the best practices for managing files in a React project.
- Organizing Your React Project
The typical structure of a React project often follows a component-based architecture. This means that your application is divided into reusable components, each with its own folder containing the necessary files (e.g., JavaScript, CSS, images, etc.).
- Navigating Through the File Structure
As your React project grows, it's essential to have a clear understanding of how to navigate through the file structure. Here are some tips:
- Use relative paths: When importing components, styles, or other files, use relative paths to reference the location within the project structure. For example, to import a Header component, you would use
import Header from '../components/Header/Header'
.
- Leverage the IDE's file explorer: Most Integrated Development Environments (IDEs) like Visual Studio Code, IntelliJ IDEA, or WebStorm provide a file explorer that allows you to easily navigate through the project's file structure. You can quickly switch between files and folders using the explorer.
- Utilize the command line: If you prefer working with the terminal, you can navigate the file structure using command-line tools like
cd
(change directory) andls
(list files and directories). - Consider using absolute paths: For larger projects, you can set up absolute path imports using a specific file in navigation directory named RoutePathConstant giving each file in the entire project a unique path. This can make your imports more readable and maintainable, especially when working with deeply nested directories.
- Employ file search: Most IDEs have built-in file search functionality that allows you to quickly find files based on their name or content. This can be especially helpful when working with a large codebase.
- Stay organized: Regularly review and refactor your file structure to ensure it remains clean and easy to navigate. As your project evolves, be mindful of keeping the file organization consistent and intuitive.
By following these best practices, you can efficiently navigate the file structure of your React project, making it easier to find, manage, and maintain your codebase.
- Example with explanation to understand the flow of a project:
- Here if we consider a to add a dummy page and render anything on it for now we can say just render "Welcome !".
- First we go to screencomponents directory in src directory and ad a new directory as dummyscreen and add in the java script file and the index file for the same.
- Render the desired output you want in dummyscreen.js export the function in it and import it in the index.js file.
- Now add a dummy directory in screen directory in src. add dummy.js and index.js files in the dummy directory.
- Import the function from dummyscreen.js into dummy.js. Export the function defined in dummy.js so as to import it into the index.js file.
- In the navigations directory in RoutePathConstant.js file define the absolute constant path to the dummy file.
- In the navigation directory in screennavigations directory make a new directory named dummyNavigation with DummyNavigation.js and index.js files in it.
- Import the path of dummy from RoutePathConstant.js in the DummyNavigation.js and then import the function in DummyNavigation.js in its index file.
- Now go to the ScreenComponents directory in the HomeScreen directory which further leads us to SideMenu directory.
- In MenuList directory which is in SideMenu add a file named DummyMenu.js and import the path of dummy from RoutePatnConstant.js.
- Now import the DummyMenu function from DummyMenu.js in the SideMenu.js file which was already present in the SideMenu directory.
- There is another file in Navigation directory which is very important that is AppRouter.js
- Import the DumyNavigation.js file in the AppRouter.js file for the changes that we have made and added to be rendered in the actual web page.
- So now all the coding part is done you can give the terminal the start npm command and observe the changes.