Logical Operators In MongoDB
MongoDB supports logical query operators. These operators are used for filtering the data and getting precise results based on the given conditions. The following table contains the comparison query operators:
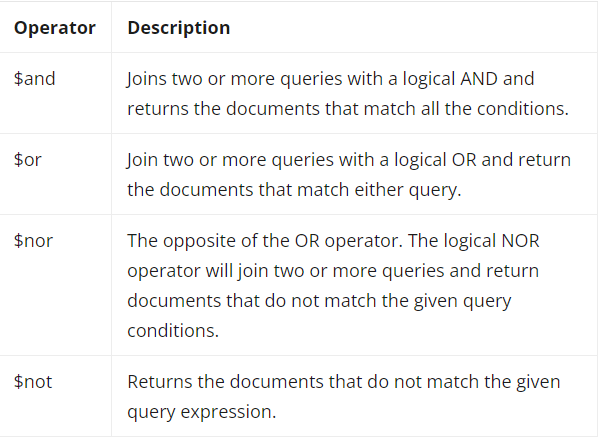
Matching values using $and operator:
In this example, we are retrieving only those employee’s documents whose status is ACTIVE and vacations is 1.
//Example
db.q_employee.find({ $and: [{"status": "ACTIVE"}, {"vacations": {$gte: 1, $lte: 3}}]})
Matching values using $nor operator:
In this example, we are retrieving only those employee’s documents whose status is not INACTIVE and whose role is not MANAGER.
//Example
db.q_employee.find({ $nor: [{"status": "INACTIVE"}, {"ROLE": "MANAGER"}]})
Matching values using $or operator:
In this example, we are retrieving only those employee’s documents whose status is ACTIVE or vacations is 1.
//Example
db.q_employee.find({ $or: [{"status": "ACTIVE"}, {"vacations": 1}]})
Matching values using $not operator:
In this example, we are retrieving only those employee’s documents whose vacations is not greater than 3.
//Example
db.q_employee.find({vacations: {$not: {$gt: 3}}})