Kotlin: Collections - List Methods
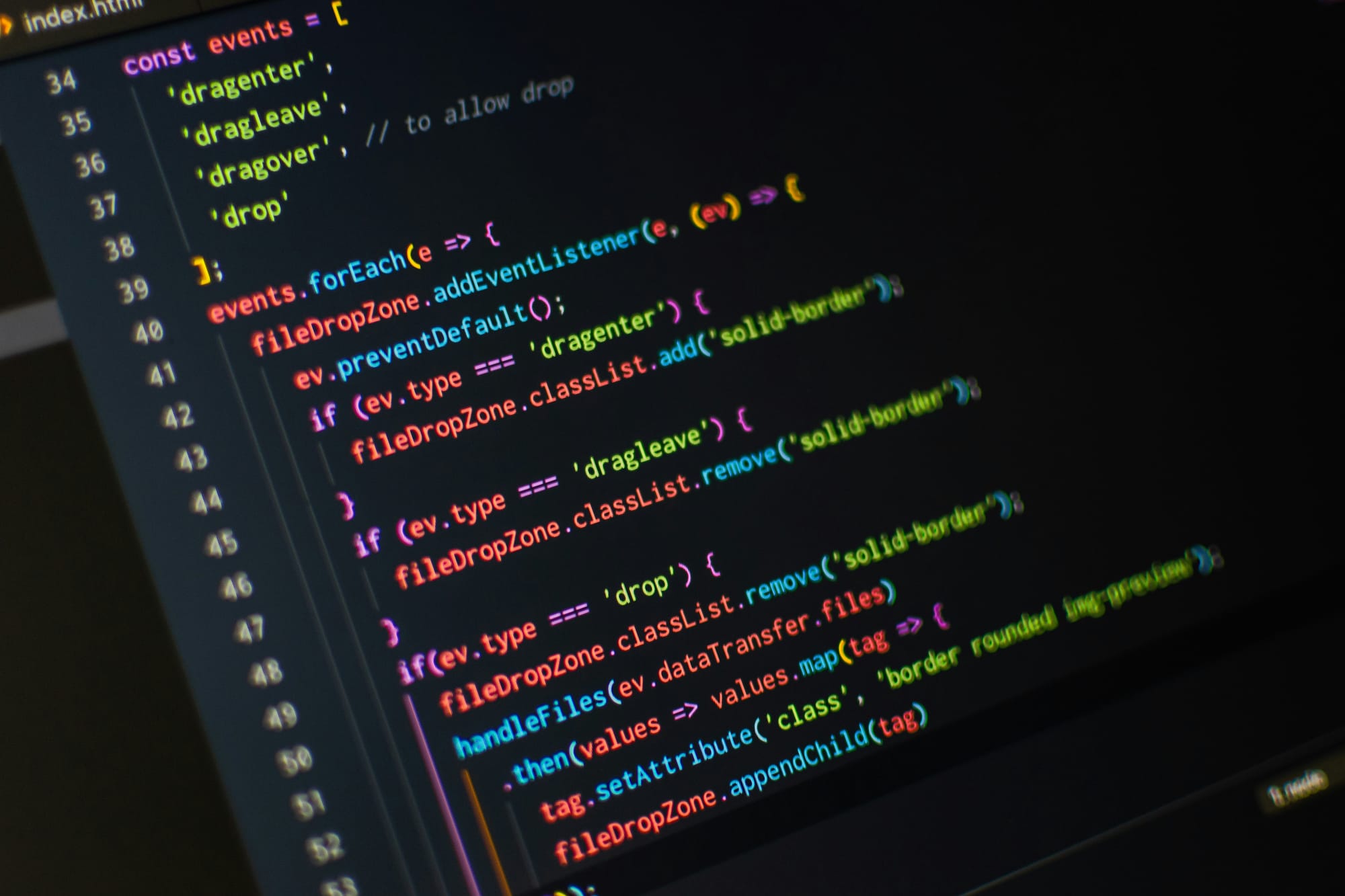
Kotlin: Collections - List Methods
List is an immutable collection in Kotlin. List allows duplicate elements, and its size cannot be changed after initialization.
1 - getOrNull() - This method is used to get the value of required index and if that value is not found or out of bound then it will print null.
Example -

In this example we have list of numbers and we used getOrNull method with index value 6, as there is no 6th index in a list. The output will be null.
Output -

2 - getOrElse() - This method is used to get the value of required index and if that index not found then it will print the value passed. In the below example we have list of numbers and we used getOrElse() method to access 7th index element from list but as there is no 7th index element in list, program will print 3.
Example -

Output -

3 - Adding Single value to list (+) - As List is immutable, we cannot directly add element to list, for adding single element as shown in below code newList is created and using (+) operator we added 8.
Example -
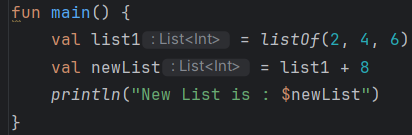
Output -

4 - Adding Multiple values to list - We can add multiple elements as shown below.
Example -
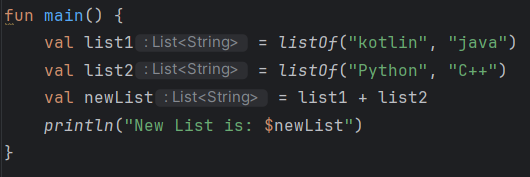
Using (+) operator "Python" and "C++" added with "kotlin" and "java" in newList.
Output -

5 - add and addAll() - New Element 4 added to mutable list and using addAll() method values 10 and 11 added to mutable list.
Example -
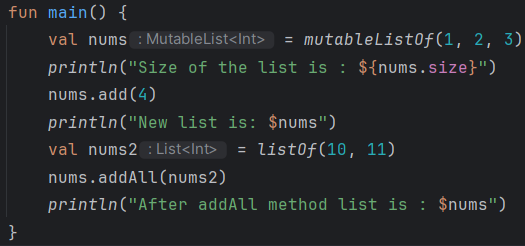
Output -

6 - Removing Element using (-) - With help of (-) Operator we can remove element from list. In below example "Mouse" is removed from list1.
Example -
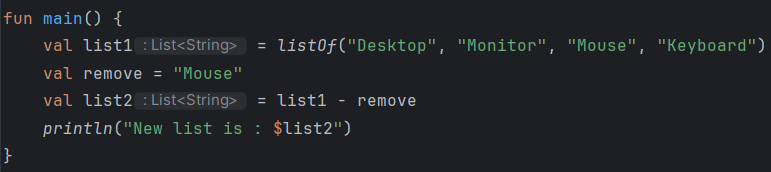
Output -

7 - removeAt() - We can remove element from particular index. Element at index 2 is 7 removed from list using removeAt() method.
Example -
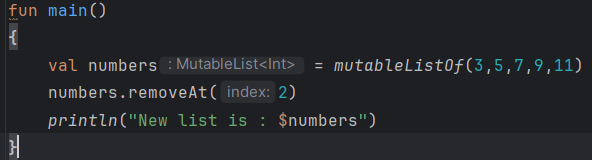
Output -
