connect()
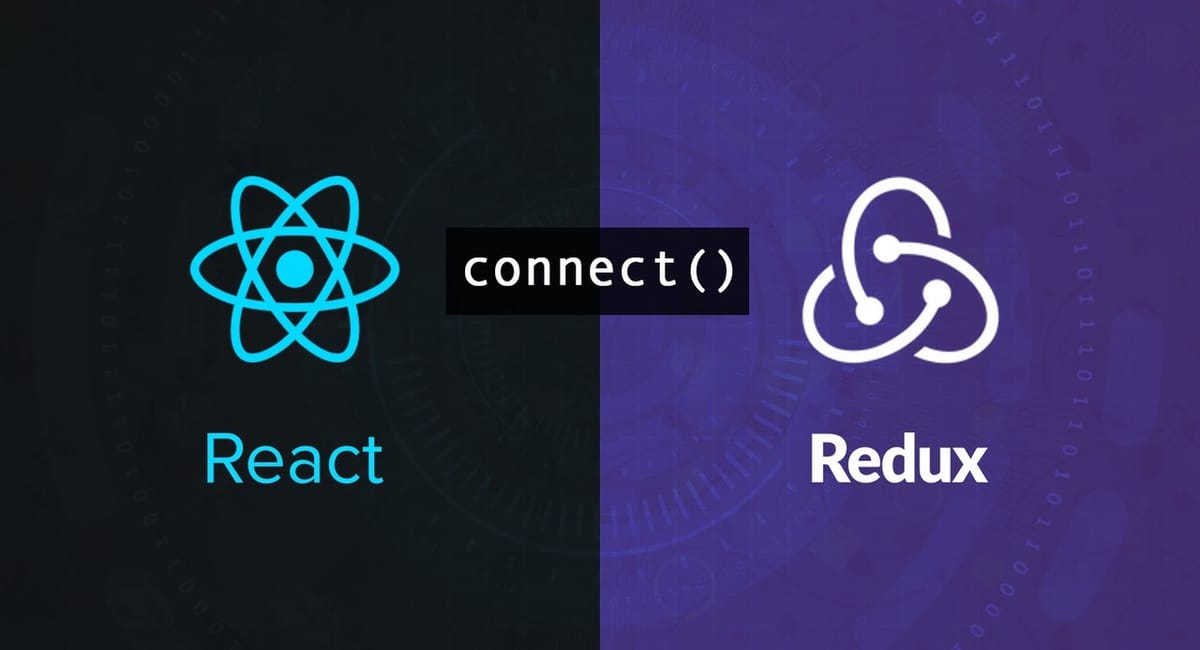
The connect()
function is redux is a way to connect React component to the Redux store. It allows the component to read data from the store and dispatch actions to the store to update the state.
It does not modify the component class passed to it; instead, it returns a new, connected component class that wraps the component you passed in.connect
accepts four different parameters, all optional. By convention, they are called:
mapStateToProps?: Function
mapDispatchToProps?: Function | Object
mergeProps?: Function
options?: Object

- Import
connect
:
First, you need to import theconnect
function fromreact-redux.

- mapStateToProps: This function specifies which parts of the state the component needs. It takes the Redux state as an argument and returns an object with the needed state data.
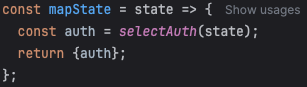
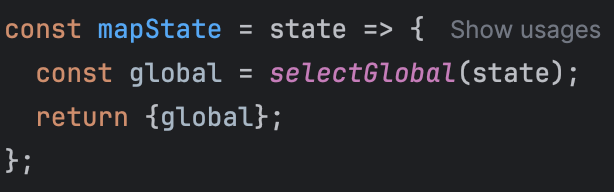
We Can Create Any Kind of Selector in Redux

null
or undefined
in place of mapStateToProps
.- mapDispatchToProps: This function specifies which actions the component can dispatch, It takes the
dispatch
function as an argument and returns an object with functions that dispatch the necessary actions.
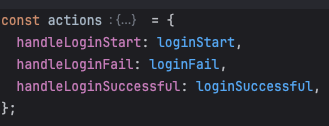
NOTE :Your component will receivedispatch
by default, i.e., when you do not supply a second parameter toconnect()
:

- mergeProps:
mergeProps?: (stateProps, dispatchProps, ownProps) => Object
This function should be specified with maximum of three parameters. They are the result ofmapStateToProps()
,mapDispatchToProps()
, and the wrapper component'sprops
, respectively: stateProps
dispatchProps
ownProps
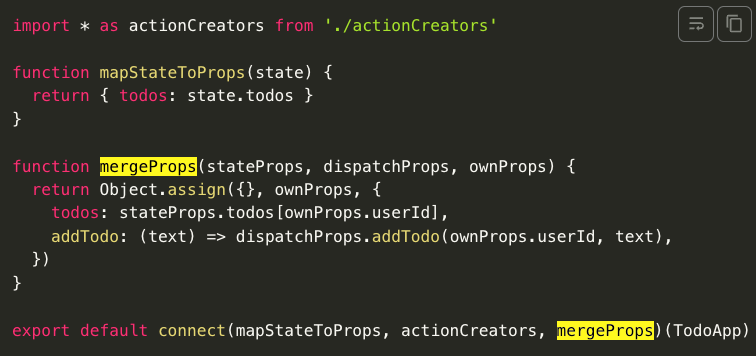
ownProps: are just the messages directly sent from the parent to the child, without going through the Redux store.
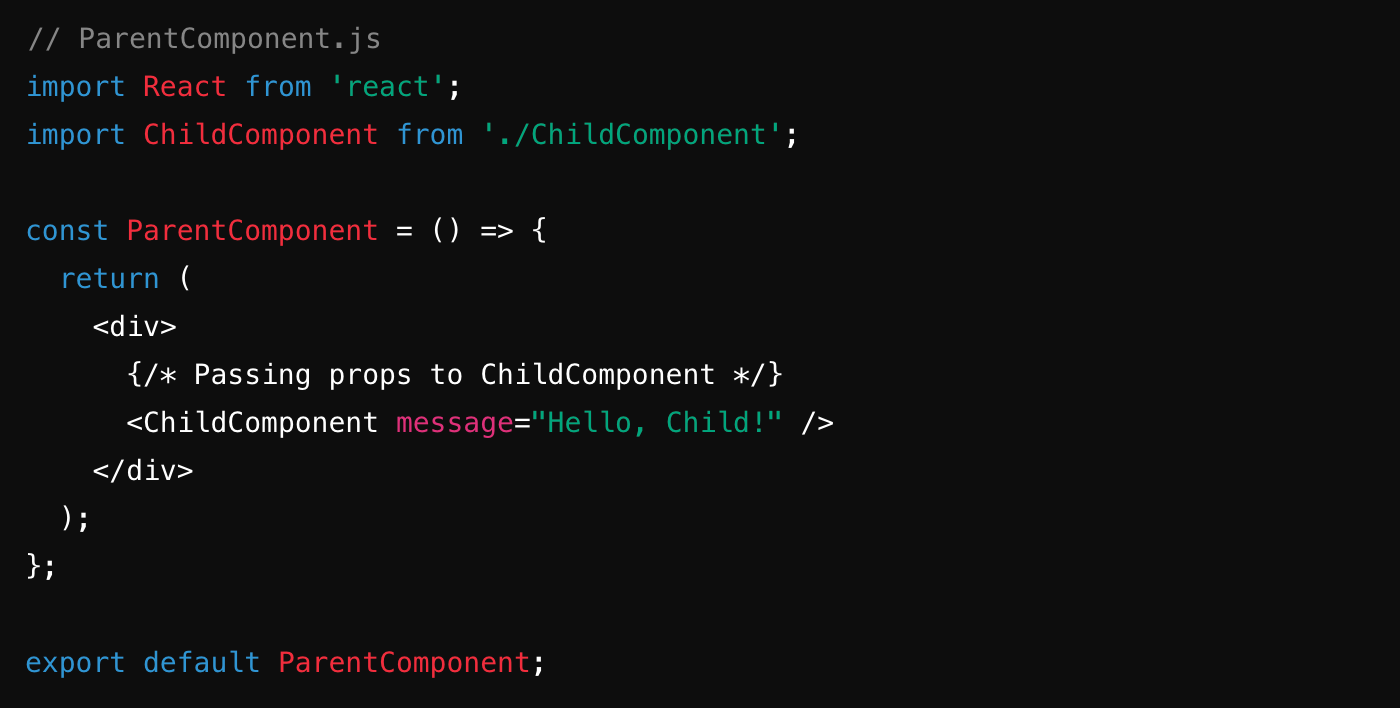
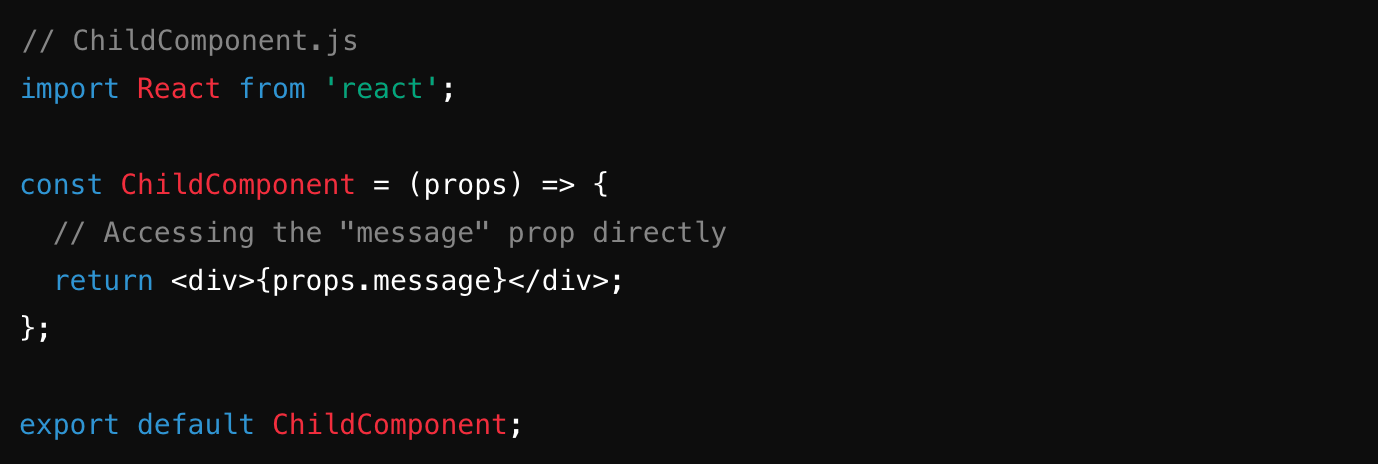
mapStateToProps: mapStateToProps?: (state, ownProps?) => Object
If your mapStateToProps
function is declared as taking two parameters, it will be called whenever the store state changes. It will be given the store state as the first parameter, and the wrapper component's props as the second parameter.

mapDispatchToProps: mapDispatchToProps?: Object | (dispatch, ownProps?) => Object
If your mapDispatchToProps
function is declared as taking two parameters, it will be called with dispatch
as the first parameter and the props passed to the wrapper component as the second parameter, and will be re-invoked whenever the connected component receives new props.
- options:
options?: Object
In Redux, theoptions
parameter isn't a built-in part of theconnect
function. Instead, it might refer to various options or settings that you can configure when creating your Redux store usingcreateStore
.
Here are some common options you might encounter when configuring a Redux store: - middleware
- reducer
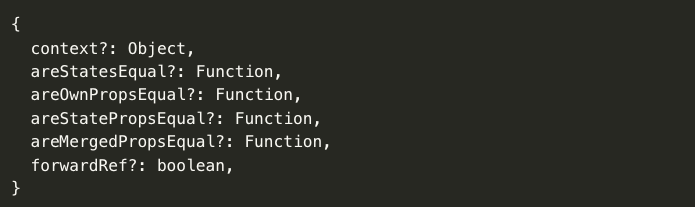
- Connect the component: You wrap your component with
connect
passing inmapStateToProps
andmapDispatchToProps
. This returns a new component that is connected to the redux store.
To know more about connect() function