Arrow Function
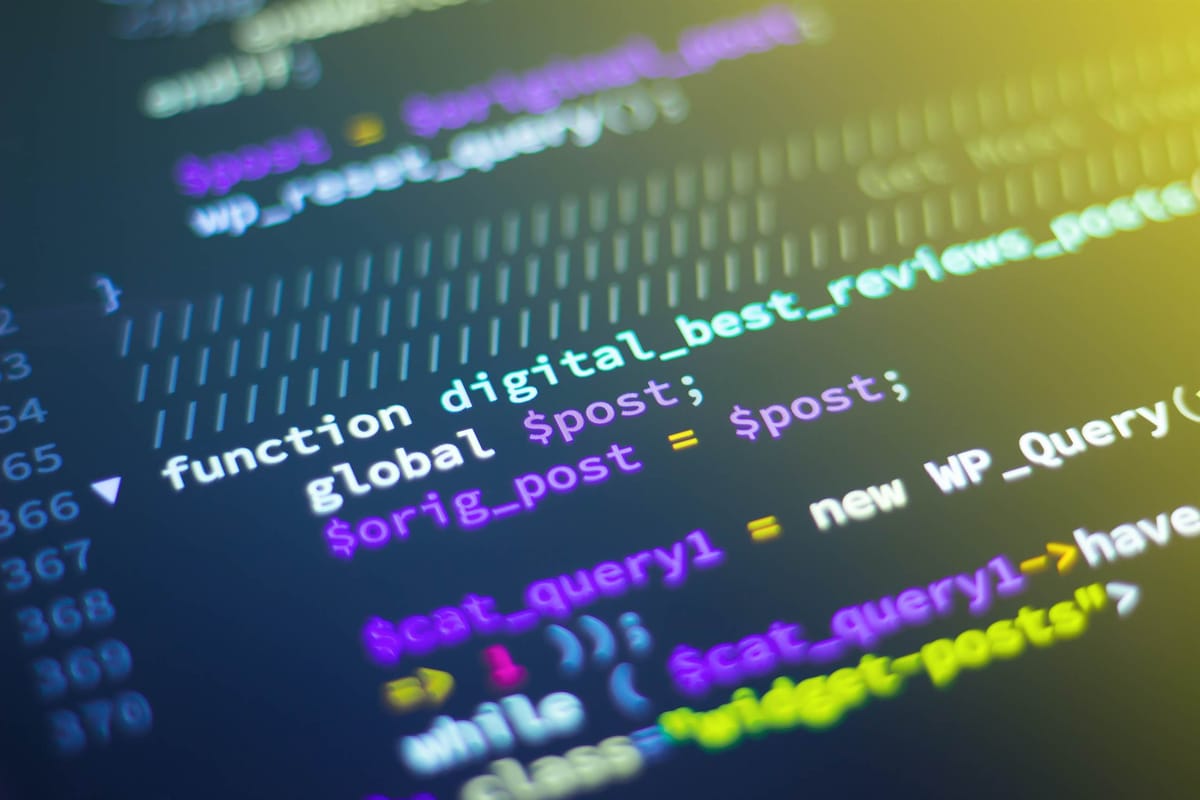
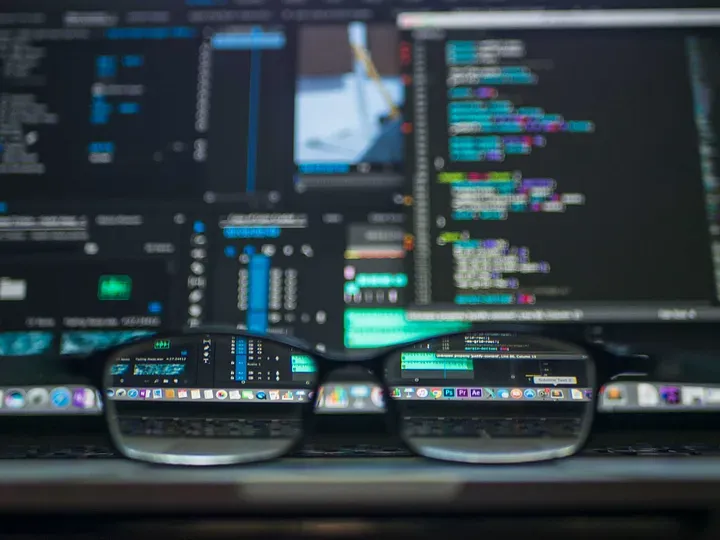
What is Arrow Function?
Arrow function {( ) =>} is concise way of writing JavaScript functions. The arrow functions in JavaScript allow us to create a shorter and anonymous function. Arrow functions were introduced in the ES6 version. They make our code more structured and readable.
Why should you use arrow functions?
1. It is a more concise way of writing functions.
2. It allows for better readability.
3. It is reduce the size of the code.
4. It is easy to understand.
Where we use arrow function?
1. Callback Functions: Arrow functions are often used as callback functions, especially in asynchronous code with methods like map, filter, and reduce.
2. Event Handlers: Arrow functions can be useful for event handler functions, where the context of this is often needed to refer to the element that triggered the event.
3. Iterating Over Arrays: Arrow functions are handy when iterating over arrays using methods like forEach.
4. Higher-Order Functions: Arrow functions are commonly used with higher-order functions, which either take other functions as arguments or return functions as their
result.
Types of Arrow Function:-
1. Single Parameter Arrow Function: When the function has only one parameter, you can omit the parentheses around the parameter list.

2. Multiple Parameters Arrow Function: When the function has multiple parameters, or if it has no parameters, you should include parentheses around the parameter list.

3. Single Line Arrow Function: When the function body consists of a single expression, you can omit the curly braces {}
and the return
keyword, and the expression's result will be automatically returned.

4. Multi-Line Arrow Function: When the function body consists of multiple statements, you need to wrap the statements in curly braces {}
. In this case, you
should include the return
keyword if you want to return a value explicitly.
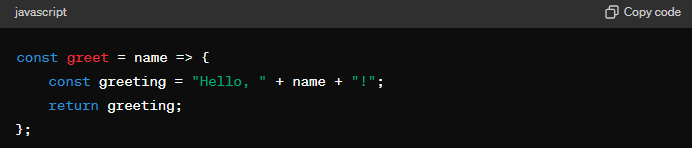
The Pieces of an Arrow Function:-
Create an arrow function by defining a variable. Then assign that variable to an anonymous arrow function.
- Function Name: a word or words to reference the function by. A variable now defines this.
- Parameters: wrapped in round brackets (). 0 or more values separated by commas to be passed into the function.
- Logic: wrapped in curly brackets {}. with an arrow symbol before the curly brackets =>. Contains the code and return value for the function.
Arrow Function Syntax-

Here,
myFunction is the name of the Function.
arg1, arg2, ...argN are the function arguments.
statement(s) is the function body.
Example 1:- Arrow Function with No Arguments
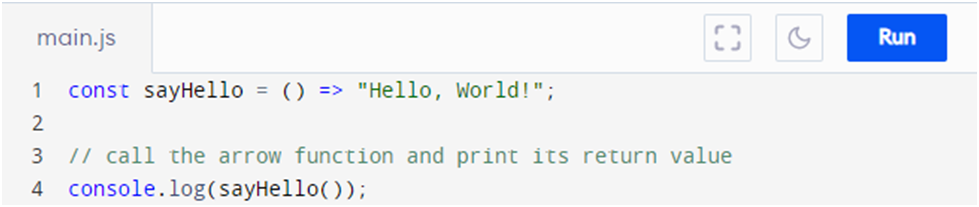
Output:-

Example 2:- Arrow Function with One Argument
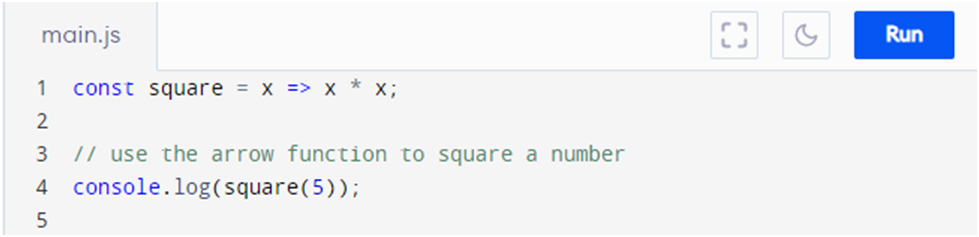
Output:-

Difference Between Arrow Function and Regular Function:-
Arrow Function-
- Syntax: (parameters) => expression or (parameters) => { statements }.
- Do not have their own this, arguments, super, or new.target bindings. Instead, they inherit these from the enclosing scope.
- Cannot be used as constructors (i.e., you cannot use new with arrow functions).
- Best suited for short, simple functions, especially when you want to maintain a shorter, more readable syntax.
- Automatically return the result of the expression if there are no curly braces {} around the function body.
Regular Function:-
- The traditional way of defining functions in JavaScript.
- Syntax: function functionName(parameters) { statements }.
- Have their own this, arguments, super, and new.target bindings.
- Can be used as constructors with the new keyword to create instances.
- More flexible and suitable for complex functions, especially those that require custom binding of this or use of arguments.